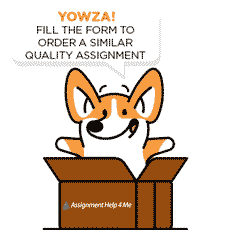

Contents
What is object oriented programming?
Object oriented programming refers to the programming paradigm based on the concept of objects that can also contain data in form of different fields and these fields are known as properties or attributes. It also includes code in the form of procedures, which are known as methods. The aim of object oriented programming is to implement Real world entities such as polymorphism, inheritance, hiding etc. It binds functions and data that operate over them in order to eliminate that no code can access this particular data instead of function. In order to learn about different object-oriented programming concepts, you can easily avail Assignment help in USA, where professionals are always available to help you in writing for the assignments related to oops.
Article Summary
This meticulous article explains in detail the crucial concept of object-oriented programming along with the multifaceted advantages and disadvantages pertaining to it. It further discusses the various fundamentals linked to the implementation of classes in the object-oriented programming language.
Advantages and Disadvantages of object oriented programming
The primary objective behind the development of object oriented approach is basically to eliminate that limitations of procedural programming method. It has proved as best approach for software development but it also includes some disadvantages. Some of the major advantages and disadvantages of OOP are as follows:
Advantages of object oriented programming (OOP)
- Object-oriented programming provides the feature of reusability of classes with the help of which it is easy to use all the classes again that have already been created previously.
- It allows for the parallel development of classes due to which object oriented programming is considered as a quickest way of development for completing the programs.
- It is one of the secure development approaches in which data is hidden that cannot be assessed by any external function.
Disadvantages of object oriented program`ming (OOP)
- It is possible that the relation among all the available classes become artificial that may cause difficulties in the development.
- In comparative to procedural approach, programs development with object oriented programming language are large in size.
- Due to large size of program, there is a need to execute more instructions.
To learn more about benefits and limitations of object oriented programming, you can easily search for best Assignment Help in USA.
Object Oriented Programming – Encapsulation, Objects and instantiation
Program Design
The application is based on a grocery shopping cart. When shopping for groceries online a Customer chooses a Product and places an Order for some quantity of it. The order is added to a Cart. A Date is set for the delivery. More orders can be added to the cart, and they can be changed up until checking out. To get to assignments in short deadlines, you can search for Instant Assignment Help. Programming experts are always available to assist you in completing your assignments on time with high- quality.
The partial UML class diagram below shows the underlying data model you should use to achieve this scenario:
Examples for implementation of classes in object oriented programming language
Product.java
This class contains the data members to store the details of a product such as product code and description etc. Also it consists of the accessors and mutators to access those data members which are declared private. Here, the concept of Encapsulation is used.
public class Product implements Comparable { protected String productCode; protected String description; protected int unitPrice; public Product(String productCode, String description, int unitPrice) { this.productCode = productCode; this.description = description; this.unitPrice = unitPrice; } public String getProductCode() { return productCode; } public void setProductCode(String productCode) { this.productCode = productCode; } public String getDescription() { return description; } public void setDescription(String description) { this.description = description; } public int getUnitPrice() { return unitPrice; } public void setUnitPrice(int unitPrice) { this.unitPrice = unitPrice; } @Override public int compareTo(Object o) { Product p=(Product)o; int mFlag = this.productCode.compareTo(p.getProductCode()); if(mFlag==0){ mFlag = this.description.compareTo(p.getDescription()); } if(mFlag==0){ mFlag = this.unitPrice-p.getUnitPrice(); } return mFlag; } } |
Customer.java
public class Customer { private String custromerId; private Name customerName; private int rewardPoints; public Customer(String custromerId,Name customerName) { this.custromerId = custromerId; this.customerName = customerName; } } |
Order.java
public class Order { private Product product; private int quantity; public Order(Product product, int quantity) { this.product = product; this.quantity = quantity; } } |
Cart.java
- The cart consists of data members for cart id delivery date and list of products ordered.
- For storing list of products we have used ArrayList class of collection.
- Cart has different methos to perform operations on cart such as findOrders(),addOrder() and removeOrder()
public class Cart { private String cartId; private Date deliveryDate; private ArrayList proOrderList; public Cart() { proOrderList = new ArrayList<>(); } public Cart(String cartId, Date deliveryDate) { this(); this.cartId = cartId; this.deliveryDate = deliveryDate; } public String getCartId() { return cartId; } public void setCartId(String cartId) { this.cartId = cartId; } public int getTotalCost() { int totalCost=0; for (Order order : proOrderList) { totalCost+=order.getCost(); } return totalCost; } public void addOrder(Order order) { proOrderList.add(order); } public boolean containsOrder(Order order) { boolean contains=false; for (Order order1 : proOrderList) { if(order.equals(order1)){ contains= true; break; } } return contains; } public void removeOrder(Order order) { boolean contains=false; for (Order order1 : proOrderList) { if(order.equals(order1)){ contains= true; break; } else{ contains= false; } } if (contains==true) { proOrderList.remove(order); System.out.println("The order has been removed from the cart"); } else { System.out.println("The order does not exist, so cannot be removed"); } } public void sortOrders() { Collections.sort(proOrderList); } public void sortOrders(Comparator comp) { Collections.sort(proOrderList,comp); } @Override public Iterator iterator() { return proOrderList.iterator(); } @Override public int compare(Order o1, Order o2) { return o1.compareTo(o2); } public Order findOrder(String productCode) { Order foundOrder = null; for (Order order : proOrderList) { Product pr = order.getProduct(); if (pr.getProductCode().equals(productCode)) { foundOrder = order; } } return foundOrder; } public ArrayList getProOrderList() { return proOrderList; } public void setProOrderList(ArrayList proOrderList) { this.proOrderList = proOrderList; } |
References
- Wegner, P. (1990). Concepts and paradigms of object-oriented programming. ACM Sigplan Oops Messenger, 1(1), 7-87.
- Stroustrup, B. (1988). What is object-oriented programming?. IEEE software, 5(3), 10-20.
- Caromel, D. (1993). Toward a method of object-oriented concurrent programming. Communications of the ACM, 36(9), 90-102.
- Campione, M., & Walrath, K. (1998). The Java Tutorial: Object-Oriented Programming for the Internet (Book/CD). Addison-Wesley Longman Publishing Co., Inc..
FAQ
what is object oriented programming in java?
Object oriented programming stands for OOP in Java. it is the programming in which the programmers are made to define the type of data of a particular set of data and the operations which stand applicable on the respective data set.
why use object oriented programming?
This is one of the most used programming techniques because of its ability to break the software into bite sized problems. This assists the programmer in solving one problem at a time reducing all the complications.
Why is OOP better than procedural programming?
OOP is considered as a better programming technique than procedural programming mostly because of the security clause provided by the first. There is no proper way to hide the data in procedural programming, which questions the security.