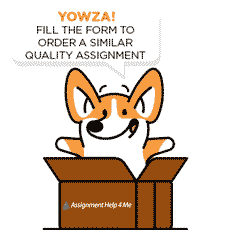

Contents
An explicit write up on Object Oriented Programming
What is object oriented programming?
Object Oriented Programming refers to the programming paradigm based on the concept of objects. It can also contain data in the form of different fields and these fields are known as properties or attributes. It also includes code in the form of procedures, which are known as methods.
The purpose of Object Oriented Programming is to implement real world entities such as polymorphism, inheritance, hiding etc. It binds functions and data that operates over them in order to ensure that no code can access the particular data instead of function. OOPs refers to the languages that utilizes the objects in programming.
Article Summary
This article defines the concept of OOPs. This write up carries out a discussion on the importance and aim of using OOPs, as a programming language. It includes various concepts such as abstraction, encapsulation, inheritance, polymorphism and many more. Moreover, OOPs concepts in Java mainly work by letting the programmers create different components, which could be re- used in several ways.
It is defined as a variety of languages that are capable of creating instances, associated with classes for the objects. It is useful for determining their type and many of the programming languages. Many of these languages are currently used by the developers to effectively support OOP.
Java is one of the main examples of OOP language that is developed around the concept of objects. It intends to improve the reusability and readability of code. This is done by describing how to structure them in a Java program in an efficient manner. In Java, OOP concepts allow the developers to create space for interactions between different objects. It also facilitates the reuse of codes without exposing them to any kind of security-related risks.
In OOP, concepts of objects and classes come into existence. The whole program is written in a class containing different objects and a number of member functions. The main reason behind the OOP is that the developers can use real-world entities in a program. Some famous languages like C++, Java, PHP, C#, Python, etc. come under Object oriented programming languages. OOP helps in applying real world entities like message passing, inheritance, polymorphism, objects, classes, abstraction, encapsulation, etc. in programming languages.
Object oriented programming concepts, principles and their workings
Different concepts of OOPs include: Polymorphism, Abstraction, Encapsulation, Inheritance, Association, Composition, Class and Method. The brief discussion about these concepts are as follows:
Polymorphism
It basically refers to the capability of OOPs programming languages to differentiate between different entities with a similar name in an efficient manner. This can be done by Java with the use of signature and declaration of entities.
Working of Polymorphism
In Java, Polymorphism mainly works with the use of reference to the parent class in order to accept the overall object in child class. In Java, Polymorphism is of two types such as:
Overloading in Java
Overriding in Java
Abstraction
The main aim of abstraction is to hide the complexity from different users and also to demonstrate the element information according to their requirements. For example: If a person wants to drive a car and doesn't want to get information regarding the internal workings. A similar statement is referred to in Java classes where an individual can easily hide internal implementation-related details with the use of abstract interfaces or classes. On the abstract level, there is a need to describe the method signatures and let all the classes implement them in an appropriate way.
Abstraction in Java is basically to hide underlying data complexity and also to avoid repetitive code. It gives the flexibility to programmers for changing the deployment of abstract behavior and also allows to achieve partial abstraction and total extraction with the use of abstract classes and interfaces.
Working of Abstraction
Abstraction in Java as an OOP concept basically Works by letting the programmers create usable and useful tools. Programmers can easily create different types of objects that can be functions, data structures, or variables. It also allows the programmers to create various classes associated with objects. There are different ways for defining these objects. For instance, a class about a variable could be an address and also specify that the address object should have street, name, zip code etc.
Encapsulation
Encapsulation is basically an approach that allows the programmers to protect all the Stored data in classes, from the system wide access. It helps in safeguarding all the internal contents from class such as real life capsulation. With the help of this, a programmer can easily deploy encapsulation in Java. This is done by considering all the fields private and by providing the public with better methods. The main aim of using encapsulation in Java is to restrict the direct access to the fields of class, and set all the fields to private.
Working of Encapsulation
It allows the programmers to re- use the functionalities without defining the security, which is also known as a powerful OOP concept because it is helpful in saving time. It allows the programmers to create code that can call the specific data from a particular database. It is also considered as very useful for reusing the code with other processes or databases. Encapsulation is basically a concept that allows a person to execute something by keeping the original data private.
Inheritance
Inheritance is basically an approach that is capable of creating the child class that effectively inherits the methods and field associated with the parent class. The child class can easily override the methods and values associated with parent class which is not very important. Inheritance in Java allows one class to extend the other class by inheriting the features. It also allows to implement DRY programming principles in an efficient manner. Adding on, it is useful to improve code reusability and multilevel inheritance in Java when a child class could have its own child class.
Syntax of Inheritance is:
class derived-class extends base-class
{
//methods and fields
}
Working of Inheritance
Inheritance is called as another labor saving OOP concept of Java that mainly works by letting an innovative class adopt the properties of others. A programmer calls the inheriting class a child class or a sub class where original class is also called as a parent class. The keyword extends could be used for defining the new class that mainly inherits the properties from the old class.
Object Oriented Programming – Encapsulation, Objects and instantiation
The application is based on a grocery shopping cart. When shopping for groceries online, a customer chooses a Product and places an Order for some quantity of it. The order is added to a Cart. A Date is set for the delivery. More orders can be added to the cart, and they can be changed up until checking out.
Examples for implementation of classes in Object Oriented Programming language
Product.java
This class contains the data members to store the details of a product such as product code and description etc. Also it consists of the accessors and mutators to access those data members which are declared private. Here, the concept of Encapsulation is used.
public class Product implements Comparable< Object >{
protected String productCode;
protected String description;
protected int unitPrice;
public Product(String productCode, String description, int unitPrice) {
this.productCode = productCode;
this.description = description;
this.unitPrice = unitPrice;
}
public String getProductCode() {
return productCode;
}
public void setProductCode(String productCode) {
this.productCode = productCode;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public int getUnitPrice() {
return unitPrice;
}
public void setUnitPrice(int unitPrice) {
this.unitPrice = unitPrice;
}
@Override
public int compareTo(Object o) {
Product p=(Product)o;
int mFlag = this.productCode.compareTo(p.getProductCode());
if(mFlag==0){
mFlag = this.description.compareTo(p.getDescription());
}
if(mFlag==0){
mFlag = this.unitPrice-p.getUnitPrice();
}
return mFlag;
}
}
Customer.java
public class Customer {
private String custromerId;
private Name customerName;
private int rewardPoints;
public Customer(String custromerId,Name customerName) {
this.custromerId = custromerId;
this.customerName = customerName;
}
}
Order.java
public class Order {
private Product product;
private int quantity;
public Order(Product product, int quantity) {
this.product = product;
this.quantity = quantity;
}
}
Advantages of Object-Oriented Programming (OOP)
The primary objective behind the development of object-oriented approaches is basically to eliminate the limitations of procedural programming methods. It has proven to be the best approach for software development. Some of the major advantages of OOP are as follows:
Object-oriented programming provides the feature of reusability of classes with the help of which, it is easy to use all the classes again that have already been created previously. Code reusability is one of the characteristics of object-oriented programming, which is done through the inheritance OOP concept. In inheritance, the class and subclasses or parent and child classes can be derived and its data member and member functions can be used. It helps in saving time and eliminates the need of doing coding again and again if similar features or functionality is required.
It allows for the parallel development of classes due to which object oriented programming is considered as the quickest way of development for completing the programs. If you are working as a programmer in a team, then you can work independently once the modular classes work out. It also allows for the relative level associated with parallel development that will not be available easily.
It is one of the secure development approaches in which data is hidden that cannot be assessed by any external function. With the use of abstraction mechanisms and Data Hiding, programmers can filter out the limited data for exposure which means that the security is maintained easily and also provides necessary data.
The coding in OOP is also very easy to maintain due to the centralized coding base, which makes it easy to create maintainable procedure code. In addition to this, it also makes it easy for keeping all the data accessible while executing and upgrading it. It is a useful approach or a process that helps in improving the security of the entire programming.
Code management in OOPs also becomes very easy as all the code is divided into a number of elements. It also allows the programmers to initialize the object variable as well as call the function easily. Therefore, easy management is also considered as a major benefit of using OOPs as a programming language.
Data redundancy is another major benefit of OOPS, which is basically a condition created where the data is stored and this similar piece of data is also shared in different places. According to this, if a user requires similar functionality in multiple classes then an individual can easily go ahead. This can be done by using common class definitions for the particular functionalities as well as inheriting them in an efficient way.
Programs are not found as disposable and the Legacy code should be dealt with on a regular basis. This in order to improve or make it capable to work with new software and computers. An object-oriented program is considered as easier for modifying and maintaining as compared to non -object oriented programs. It requires more hard work and efforts to write the program in OOPs to complete the work efficiently.
Disadvantages of object oriented programming (OOP)
It is possible that the relation among all the available classes become artificial that may cause difficulties in the development.
In comparison to the procedural approach, programs developed with Object-Oriented Programming language are large in size. Not only this, it requires additional resources for its implementation which can lead to runtime overhead issues.
The concepts included in Object-Oriented Programming are a bit more complex than the procedural programming languages. Therefore, it might become difficult for programmers or coders to understand their complex working in the initial development phase. For instance- concepts of polymorphism, abstraction, and inheritance are a little hard to comprehend. Therefore, it can be said that programming using Object Oriented requires a specialized skill set in developing program logic. Otherwise, it may become difficult for programmers to manage and debug the code.
The increased line of code will have a significant impact on the processing time. This is because of the instructions that are added in the program logic and thereby these instructions will require comparatively more processing time for its execution.
Majority of programs created using the Object Oriented model are more efficient and flexible in nature, yet there are some limitations to it. One such limitation is the inability of these programs to fit in every situation. In such cases, other programming languages such as functional or other procedural programming languages are more suitable to solve such problems.
There is another functionality of message passing in OOP methodology which enables objects of different classes to share data using message passing techniques. However, in the case of complex software applications, it might be a challenging task to track messages among different instances. Thus, it increases overall processing time in debugging of software modules.
Due to the large size of the program, there is a need to execute more instructions.
Alternatives to Object Oriented Programming
Object Oriented programming has gained a lot of popularity because of its easy explanation with which a programmer feels sophisticated to work with. Along with this, there are several other alternatives to Object Oriented Programming, which can be used by the programmers for writing a code. There is an example of a Pen class and a Paper class. Both of these classes easily inherit from Material base class. In this, there is a pointer to Material that is pointing to an instance of a pen or a paper. Complete coding for this is elaborated below:
#include
#include
class Material {
public:
virtual void speak() = 0;
};
class Pen : Material {
public:
void speak() { std::cout << “Paper”; }
};
class Paper: Material {
public:
void speak() { std::cout << “bark”; }
};
int main() {
Material * Material;
// Use current system time as a random number
if (time(0)%2) Material = new Cat();
else Material = new paper();
Material ->speak();
return 0;
}
Procedurally Oriented Programming
It is basically a programming paradigm that derived from structured programming. It is based on the concept associated with procedure calls where procedures are known as functions, routines or subroutines that contain a series of computational steps which are to be carried out. The code for a pen and a paper is written below:
#include
#include
int main() {
if (time(0)%2) std::cout << “pen”;
else std::cout << “paper”;
return 0;
}
Data Oriented Programming
Lisp is one of the main examples of data oriented. In this particular paradigm, all the things are considered as data including the code. Rather than writing the sequence of steps as done in other paradigms, there is a need of describing the data only.
Lisp, the main example of DOP, basically stands for LISt processing, which is actually a family of different programming languages that emphasizes data driven programming. This type of programming is also concerned with the utilisation of data in order to evaluate the way in which it organises data. This may have a major impact on the performance. The use of all these variables in memory is called data locality.
Data Driven Design
This particular approach is all about determining the behaviour of a program through data instead of code. It is a design method in which the structure of a software system reflects the overall structure of data which is processed by the system. Data driven design is done with the use of information gathered from qualitative and quantitative sources in order to inform how to make the decisions for a number of users. Some common tools that are useful for collecting data are site usage, consumer research, discovery calls etc.
Conclusion
The given article concludes that OOPs is one of the main topics in programming languages. A programmer must have complete knowledge regarding this concept in order to perform programming in an efficient manner. This article provides different types of information such as advantages and disadvantages of OOPs and other important concepts related to it.
FAQ
What is the best way to learn object oriented programming?
The best way to learn object oriented programming is to join a program which helps in understanding the course in detail. A proper course in the same would help the students in having a detailed understanding of the subject with the help of a qualified instructor.
What are classes in object oriented programming?
The various classes present in object oriented programming include Object classes, Mixing classes, Abstract classes and Metaclasses.
Why do we study object oriented programming?
Object oriented programming is a well structured field of study, which helps the programmers bind the data together and make them aware of various functions. These also aids in modulating for easy troubleshooting.